Procedural Meshes
Project Type: Self-Study
Software Used: Unreal Engine 5
Language Used: C++
Primary Role: Gameplay Programmer
Project Summary
In six months, I constructed a mesh cutting library for Unreal Engine 5 with C++. A user can draw any shape onto a wall and remove that shape from the mesh. As the mesh is cut, a suite of custom debugging tools displays how each part of the algorithm works.
My core goals going into the project were:
- Decoupled and clean code
- Test Driven
- And to learn about rendering meshes
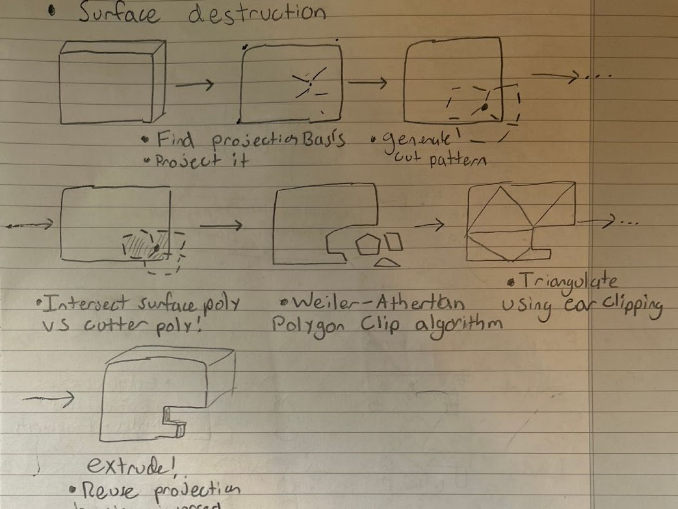
Work Highlights
Triangulation of Polygons
One core aspect of the project is taking a 2D polygon and converting it into a renderable mesh.
I accomplish this in three steps:
- Triangulate the 2D Polygon
- Store the triangles into an array of points and tris for rendering
- Add the side faces
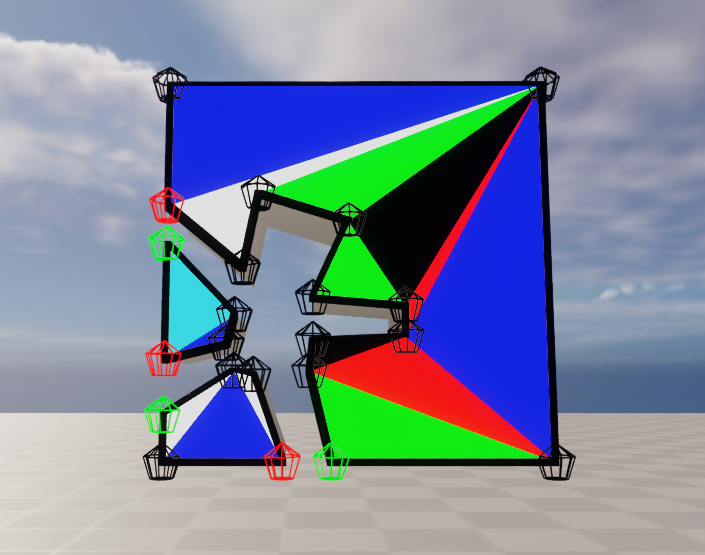
To triangulate I utilized the Ear-Clipping algorithm. Which simply loops through vertices on our polygon, sees if their neighbor vertices form a valid triangle, and if so clips the new triangle from the polygon.
A valid triangle must be convex, have no vertices inside of it, and be clockwise for rendering winding order.
Below is my code for the triangulation step, and a link to it on my GitHub repo.
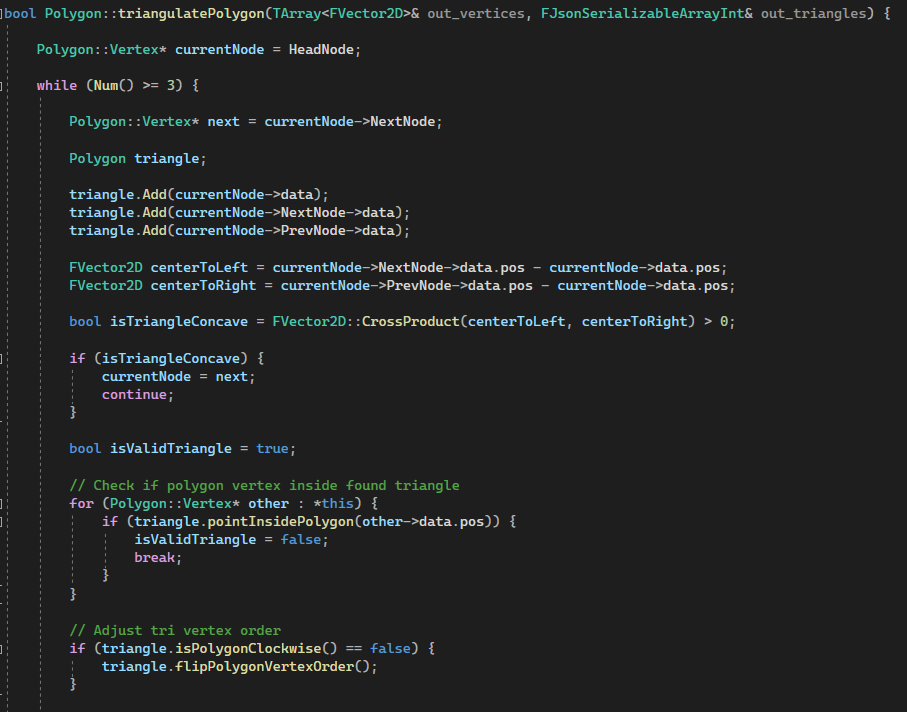
Decoupled Logic
To separate the logic of the Polygon cutting steps and mesh rendering steps, I built two core classes Polygon.cpp and WallCutter.cpp.
To further decouple these two systems, Polygon.cpp prevents users from having responsibility over Node instantiation and destruction. Users can only pass a struct to the Polygon vertex constructor. This data is then used to initialize a vertex class tracked internally in Polygon.cpp.
Below is my Polygon class hierarchy and a link to it on my GitHub repo. Note: VertexData is our public struct for users, Vertex is the internal wrapper class with extra data for a Linked List.
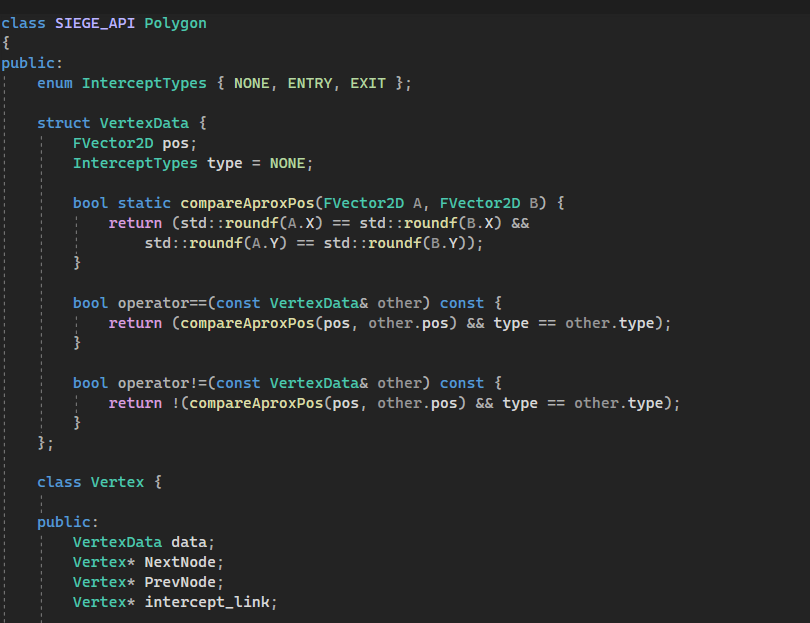
Test System
My core testing is broken up into two parts: Unit Tests and an In-game step-through debugger. Shown below are my 16 unit tests, each targeting a different aspect of the Polygon.cpp and WallCutter.cpp classes.
Below are my Unit Tests in Unreal Engines test viewer. (13 Polygon tests, 3 WallCutter tests)
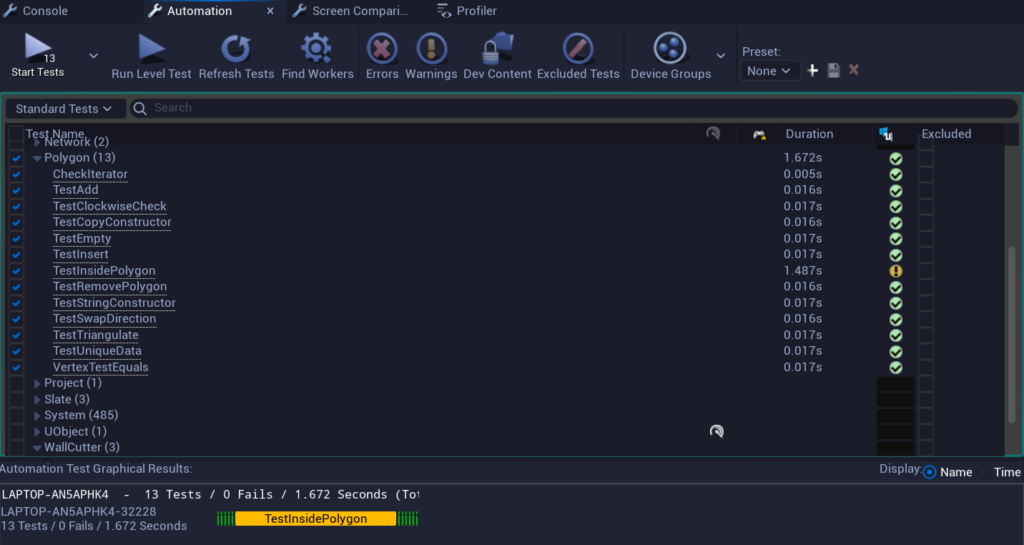
To assist unit tests I built a string constructor for Polygon class instances. Instead of long polygon constructors, the test scripts are simplified to simple strings.
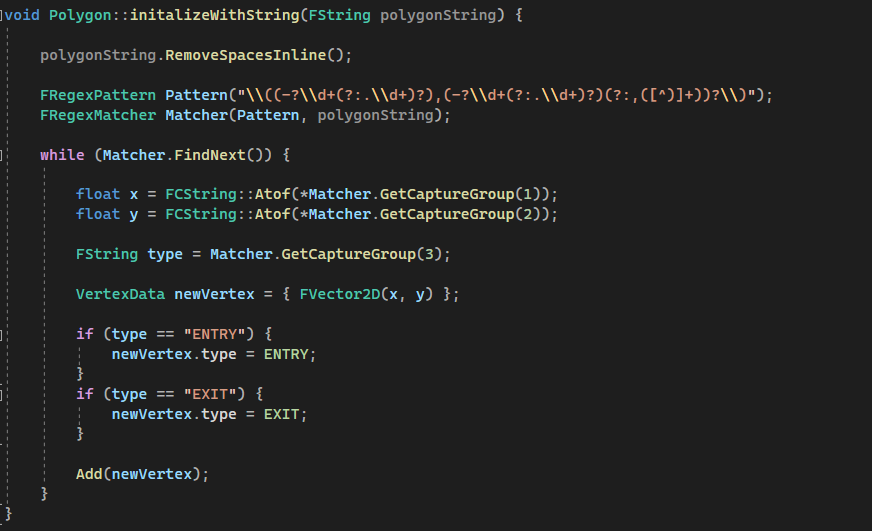
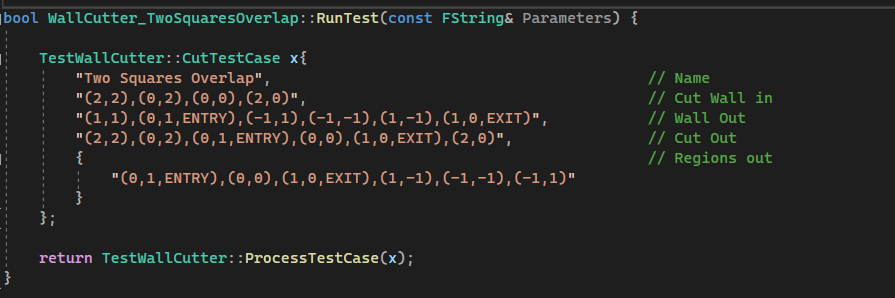
Finally, my in-game debugger steps through the execution of each step of my design every second. This allowed me to catch any errors between functions live. In the first video, this is showcased.
Contact Me!
Reach out at jonahpryan@gmail.com or in/jonah-ryan